Statistics using SimpleITK
Contents
Statistics using SimpleITK#
We can use Simple for extracting features from label images. For convenience reasons we use the napari-simpleitk-image-processing library.
import numpy as np
import pandas as pd
from skimage.io import imread
import pyclesperanto_prototype as cle
from napari_simpleitk_image_processing import watershed_otsu_labeling
from napari_simpleitk_image_processing import label_statistics
blobs = imread('../../data/blobs.tif')
cle.imshow(blobs)
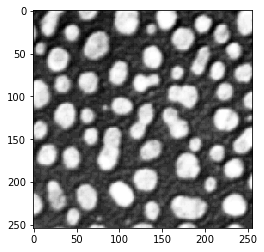
Starting point: a segmented label image#
labels = watershed_otsu_labeling(blobs)
cle.imshow(labels, labels=True)
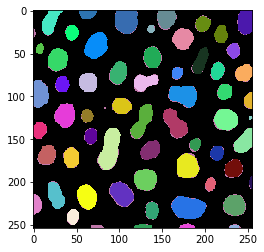
Label statistics#
statistics = label_statistics(blobs, labels,
intensity=True,
size=True,
shape=True,
perimeter=True,
position=True,
moments=True)
df = pd.DataFrame(statistics)
df
label | maximum | mean | median | minimum | sigma | sum | variance | bbox_0 | bbox_1 | ... | number_of_pixels_on_border | perimeter | perimeter_on_border | perimeter_on_border_ratio | principal_axes0 | principal_axes1 | principal_axes2 | principal_axes3 | principal_moments0 | principal_moments1 | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
0 | 1 | 224.0 | 137.526132 | 136.0 | 112.0 | 13.360739 | 157880.0 | 178.509343 | 0 | 0 | ... | 36 | 2461.579651 | 36.0 | 0.014625 | -0.574118 | -0.818773 | 0.818773 | -0.574118 | 5063.911496 | 5560.184284 |
1 | 2 | 232.0 | 193.014354 | 200.0 | 128.0 | 28.559077 | 80680.0 | 815.620897 | 11 | 0 | ... | 16 | 85.499572 | 16.0 | 0.187135 | 0.902494 | 0.430703 | -0.430703 | 0.902494 | 17.056706 | 72.884853 |
2 | 3 | 224.0 | 179.846995 | 184.0 | 128.0 | 21.328889 | 32912.0 | 454.921516 | 53 | 0 | ... | 21 | 53.456120 | 21.0 | 0.392846 | -0.042759 | -0.999085 | 0.999085 | -0.042759 | 8.637199 | 27.432794 |
3 | 4 | 248.0 | 207.082171 | 216.0 | 120.0 | 27.772832 | 133568.0 | 771.330194 | 95 | 0 | ... | 23 | 93.409370 | 23.0 | 0.246228 | 0.991601 | 0.129334 | -0.129334 | 0.991601 | 48.975064 | 55.851742 |
4 | 5 | 248.0 | 223.146402 | 232.0 | 128.0 | 30.246515 | 89928.0 | 914.851647 | 144 | 0 | ... | 19 | 74.218143 | 19.0 | 0.256002 | 0.974707 | 0.223487 | -0.223487 | 0.974707 | 32.059800 | 33.765222 |
5 | 6 | 248.0 | 214.906725 | 224.0 | 128.0 | 26.386796 | 99072.0 | 696.263020 | 238 | 0 | ... | 39 | 80.787183 | 40.0 | 0.495128 | 0.999408 | 0.034407 | -0.034407 | 0.999408 | 23.320204 | 59.820502 |
6 | 7 | 248.0 | 211.565891 | 224.0 | 136.0 | 30.197236 | 54584.0 | 911.873073 | 189 | 7 | ... | 0 | 57.938471 | 0.0 | 0.000000 | 0.932037 | 0.362364 | -0.362364 | 0.932037 | 18.705896 | 22.720115 |
7 | 8 | 200.0 | 166.171429 | 168.0 | 136.0 | 16.466894 | 11632.0 | 271.158592 | 133 | 17 | ... | 0 | 29.917295 | 0.0 | 0.000000 | 0.960385 | 0.278678 | -0.278678 | 0.960385 | 4.741825 | 6.584094 |
8 | 9 | 224.0 | 176.932331 | 176.0 | 128.0 | 24.022064 | 47064.0 | 577.059555 | 211 | 17 | ... | 0 | 59.834590 | 0.0 | 0.000000 | 0.997651 | 0.068495 | -0.068495 | 0.997651 | 14.969363 | 30.395186 |
9 | 10 | 240.0 | 191.598174 | 200.0 | 128.0 | 28.239851 | 41960.0 | 797.489171 | 37 | 18 | ... | 0 | 53.360835 | 0.0 | 0.000000 | 0.992904 | 0.118917 | -0.118917 | 0.992904 | 15.331341 | 19.871929 |
10 | 11 | 232.0 | 190.962963 | 200.0 | 128.0 | 23.617106 | 92808.0 | 557.767698 | 162 | 21 | ... | 0 | 79.485892 | 0.0 | 0.000000 | 0.950824 | 0.309732 | -0.309732 | 0.950824 | 35.879673 | 42.001599 |
11 | 12 | 232.0 | 173.000000 | 176.0 | 128.0 | 19.441981 | 110720.0 | 377.990610 | 59 | 26 | ... | 0 | 93.218800 | 0.0 | 0.000000 | 0.848443 | 0.529287 | -0.529287 | 0.848443 | 38.069371 | 69.135089 |
12 | 13 | 200.0 | 168.351648 | 168.0 | 128.0 | 19.226356 | 15320.0 | 369.652747 | 3 | 39 | ... | 0 | 35.050291 | 0.0 | 0.000000 | 0.945102 | 0.326774 | -0.326774 | 0.945102 | 5.568379 | 9.510959 |
13 | 14 | 248.0 | 203.019608 | 208.0 | 128.0 | 32.682072 | 41416.0 | 1068.117840 | 226 | 39 | ... | 0 | 51.464716 | 0.0 | 0.000000 | 0.999604 | -0.028149 | 0.028149 | 0.999604 | 13.550254 | 19.604062 |
14 | 15 | 248.0 | 216.156863 | 224.0 | 136.0 | 28.644305 | 88192.0 | 820.496218 | 129 | 42 | ... | 0 | 72.226739 | 0.0 | 0.000000 | 0.985169 | -0.171585 | 0.171585 | 0.985169 | 26.472262 | 39.901074 |
15 | 16 | 232.0 | 181.963255 | 184.0 | 120.0 | 24.411574 | 69328.0 | 595.924962 | 185 | 43 | ... | 0 | 81.382011 | 0.0 | 0.000000 | 0.925427 | 0.378925 | -0.378925 | 0.925427 | 12.588614 | 76.471615 |
16 | 17 | 248.0 | 197.333333 | 200.0 | 120.0 | 26.311828 | 97088.0 | 692.312288 | 17 | 44 | ... | 0 | 79.485892 | 0.0 | 0.000000 | 0.949997 | 0.312260 | -0.312260 | 0.949997 | 33.707850 | 45.675040 |
17 | 18 | 248.0 | 200.160401 | 208.0 | 128.0 | 27.596051 | 79864.0 | 761.542046 | 90 | 60 | ... | 0 | 72.782100 | 0.0 | 0.000000 | 0.959680 | 0.281094 | -0.281094 | 0.959680 | 21.950618 | 46.037485 |
18 | 19 | 248.0 | 205.943775 | 216.0 | 136.0 | 30.086114 | 51280.0 | 905.174245 | 207 | 61 | ... | 0 | 57.153073 | 0.0 | 0.000000 | 0.954815 | 0.297199 | -0.297199 | 0.954815 | 14.780837 | 26.562206 |
19 | 20 | 232.0 | 192.235988 | 200.0 | 120.0 | 25.416595 | 65168.0 | 646.003316 | 235 | 63 | ... | 11 | 66.863705 | 11.0 | 0.164514 | -0.593398 | -0.804909 | 0.804909 | -0.593398 | 22.740379 | 32.242766 |
20 | 21 | 224.0 | 189.577465 | 200.0 | 136.0 | 24.291178 | 26920.0 | 590.061333 | 162 | 66 | ... | 0 | 42.864805 | 0.0 | 0.000000 | 0.946821 | 0.321762 | -0.321762 | 0.946821 | 9.729421 | 13.167326 |
21 | 22 | 224.0 | 187.870324 | 192.0 | 128.0 | 24.658125 | 75336.0 | 608.023142 | 53 | 73 | ... | 0 | 71.996702 | 0.0 | 0.000000 | 0.993149 | -0.116857 | 0.116857 | 0.993149 | 28.718371 | 35.697584 |
22 | 23 | 248.0 | 208.960836 | 216.0 | 128.0 | 30.936449 | 80032.0 | 957.063907 | 117 | 76 | ... | 0 | 79.485892 | 0.0 | 0.000000 | -0.249601 | -0.968349 | 0.968349 | -0.249601 | 17.353521 | 61.813901 |
23 | 24 | 240.0 | 182.081301 | 184.0 | 128.0 | 26.433619 | 44792.0 | 698.736220 | 26 | 77 | ... | 0 | 56.597713 | 0.0 | 0.000000 | 0.999988 | -0.004899 | 0.004899 | 0.999988 | 16.419754 | 23.402458 |
24 | 25 | 248.0 | 199.629032 | 200.0 | 128.0 | 27.426587 | 99016.0 | 752.217660 | 0 | 82 | ... | 29 | 84.484136 | 29.0 | 0.343260 | 0.998757 | -0.049841 | 0.049841 | 0.998757 | 24.491079 | 67.691848 |
25 | 26 | 240.0 | 192.449438 | 192.0 | 120.0 | 26.732458 | 51384.0 | 714.624314 | 214 | 86 | ... | 0 | 58.493832 | 0.0 | 0.000000 | 0.929427 | 0.369006 | -0.369006 | 0.929427 | 18.770857 | 24.067028 |
26 | 27 | 240.0 | 202.691706 | 208.0 | 128.0 | 24.550960 | 129520.0 | 602.749633 | 158 | 88 | ... | 0 | 97.891721 | 0.0 | 0.000000 | 0.252109 | -0.967699 | 0.967699 | 0.252109 | 34.790068 | 80.474893 |
27 | 28 | 232.0 | 200.641975 | 208.0 | 136.0 | 22.958406 | 32504.0 | 527.088413 | 245 | 95 | ... | 20 | 51.004641 | 20.0 | 0.392121 | 0.999016 | 0.044348 | -0.044348 | 0.999016 | 7.500566 | 24.475429 |
28 | 29 | 232.0 | 201.661721 | 208.0 | 136.0 | 24.593688 | 67960.0 | 604.849513 | 92 | 102 | ... | 0 | 66.308345 | 0.0 | 0.000000 | 0.159256 | -0.987237 | 0.987237 | 0.159256 | 22.243114 | 32.490995 |
29 | 30 | 240.0 | 202.541910 | 208.0 | 136.0 | 26.036533 | 103904.0 | 677.901072 | 26 | 108 | ... | 0 | 82.167409 | 0.0 | 0.000000 | 0.989629 | 0.143647 | -0.143647 | 0.989629 | 32.933086 | 51.101812 |
30 | 31 | 232.0 | 192.649396 | 200.0 | 120.0 | 25.700290 | 111544.0 | 660.504892 | 113 | 109 | ... | 0 | 105.610950 | 0.0 | 0.000000 | 0.903873 | 0.427801 | -0.427801 | 0.903873 | 18.013291 | 134.834719 |
31 | 32 | 248.0 | 195.953871 | 200.0 | 120.0 | 30.668210 | 118944.0 | 940.539123 | 151 | 114 | ... | 0 | 99.692555 | 0.0 | 0.000000 | 0.830015 | -0.557742 | 0.557742 | 0.830015 | 25.231170 | 101.168109 |
32 | 33 | 232.0 | 184.131148 | 192.0 | 128.0 | 26.165665 | 33696.0 | 684.642046 | 56 | 115 | ... | 0 | 49.568597 | 0.0 | 0.000000 | 0.991750 | -0.128190 | 0.128190 | 0.991750 | 12.926033 | 16.531401 |
33 | 34 | 248.0 | 215.125448 | 224.0 | 128.0 | 30.288772 | 120040.0 | 917.409728 | 211 | 115 | ... | 0 | 85.174249 | 0.0 | 0.000000 | 0.220924 | -0.975291 | 0.975291 | 0.220924 | 40.823045 | 48.505271 |
34 | 35 | 176.0 | 151.529412 | 152.0 | 136.0 | 15.091875 | 2576.0 | 227.764706 | 251 | 124 | ... | 2 | 14.843629 | 2.0 | 0.134738 | -0.163228 | -0.986588 | 0.986588 | -0.163228 | 1.046852 | 1.755916 |
35 | 36 | 232.0 | 191.224806 | 200.0 | 120.0 | 25.409101 | 49336.0 | 645.622417 | 0 | 130 | ... | 13 | 57.153073 | 13.0 | 0.227459 | 0.970628 | 0.240586 | -0.240586 | 0.970628 | 16.246640 | 26.130982 |
36 | 37 | 248.0 | 201.665497 | 200.0 | 128.0 | 28.167372 | 172424.0 | 793.400857 | 76 | 137 | ... | 0 | 121.660584 | 0.0 | 0.000000 | 0.975948 | 0.218005 | -0.218005 | 0.975948 | 27.394927 | 179.878327 |
37 | 38 | 248.0 | 208.017467 | 208.0 | 128.0 | 29.009010 | 95272.0 | 841.522670 | 233 | 137 | ... | 23 | 83.278130 | 23.0 | 0.276183 | 0.844799 | 0.535083 | -0.535083 | 0.844799 | 21.736051 | 65.950501 |
38 | 39 | 232.0 | 197.419355 | 208.0 | 128.0 | 28.666250 | 42840.0 | 821.753883 | 60 | 138 | ... | 0 | 52.805475 | 0.0 | 0.000000 | 0.986995 | 0.160753 | -0.160753 | 0.986995 | 14.052349 | 21.247148 |
39 | 40 | 232.0 | 191.774648 | 200.0 | 136.0 | 24.532044 | 27232.0 | 601.821197 | 183 | 149 | ... | 0 | 42.309444 | 0.0 | 0.000000 | 0.965804 | 0.259275 | -0.259275 | 0.965804 | 9.197500 | 13.952669 |
40 | 41 | 232.0 | 180.952381 | 184.0 | 128.0 | 24.471401 | 72200.0 | 598.849486 | 125 | 152 | ... | 0 | 72.226739 | 0.0 | 0.000000 | -0.653319 | -0.757082 | 0.757082 | -0.653319 | 22.888892 | 44.383172 |
41 | 42 | 248.0 | 204.708333 | 216.0 | 120.0 | 28.500531 | 78608.0 | 812.280244 | 6 | 157 | ... | 0 | 70.100583 | 0.0 | 0.000000 | 0.904586 | 0.426291 | -0.426291 | 0.904586 | 25.066435 | 37.439939 |
42 | 43 | 248.0 | 191.221239 | 192.0 | 128.0 | 26.451712 | 43216.0 | 699.693058 | 206 | 158 | ... | 0 | 53.916196 | 0.0 | 0.000000 | 0.977778 | 0.209643 | -0.209643 | 0.977778 | 15.830580 | 20.544723 |
43 | 44 | 240.0 | 200.091691 | 208.0 | 136.0 | 27.115603 | 69832.0 | 735.255937 | 36 | 160 | ... | 0 | 66.863705 | 0.0 | 0.000000 | 0.999560 | -0.029663 | 0.029663 | 0.999560 | 21.238298 | 36.355055 |
44 | 45 | 240.0 | 204.294599 | 216.0 | 128.0 | 28.702058 | 124824.0 | 823.808151 | 167 | 167 | ... | 0 | 88.736449 | 0.0 | 0.000000 | 0.984212 | -0.176992 | 0.176992 | 0.984212 | 44.044878 | 54.215343 |
45 | 46 | 248.0 | 215.719298 | 224.0 | 136.0 | 32.074924 | 73776.0 | 1028.800741 | 223 | 174 | ... | 0 | 65.752985 | 0.0 | 0.000000 | 0.756607 | 0.653870 | -0.653870 | 0.756607 | 24.162673 | 30.794348 |
46 | 47 | 240.0 | 205.387454 | 216.0 | 136.0 | 24.250565 | 111320.0 | 588.089898 | 117 | 185 | ... | 0 | 83.833491 | 0.0 | 0.000000 | 0.116985 | -0.993134 | 0.993134 | 0.116985 | 41.327753 | 45.322011 |
47 | 48 | 232.0 | 194.711864 | 200.0 | 136.0 | 29.510402 | 11488.0 | 870.863822 | 251 | 193 | ... | 15 | 33.479495 | 15.0 | 0.448035 | 0.999821 | 0.018944 | -0.018944 | 0.999821 | 1.690956 | 14.325132 |
48 | 49 | 232.0 | 186.684211 | 192.0 | 128.0 | 25.196557 | 28376.0 | 634.866504 | 200 | 195 | ... | 0 | 44.435601 | 0.0 | 0.000000 | 0.980620 | 0.195921 | -0.195921 | 0.980620 | 9.610562 | 15.277380 |
49 | 50 | 232.0 | 168.875000 | 168.0 | 120.0 | 25.410148 | 75656.0 | 645.675615 | 18 | 200 | ... | 0 | 82.262694 | 0.0 | 0.000000 | 0.958423 | -0.285352 | 0.285352 | 0.958423 | 17.922660 | 72.404250 |
50 | 51 | 248.0 | 229.430657 | 248.0 | 144.0 | 26.958373 | 125728.0 | 726.753866 | 90 | 200 | ... | 0 | 85.078964 | 0.0 | 0.000000 | 0.774700 | -0.632329 | 0.632329 | 0.774700 | 31.162401 | 61.364008 |
51 | 52 | 216.0 | 191.100952 | 200.0 | 128.0 | 22.709233 | 100328.0 | 515.709255 | 51 | 203 | ... | 0 | 83.278130 | 0.0 | 0.000000 | 0.957483 | -0.288491 | 0.288491 | 0.957483 | 31.380235 | 55.869804 |
52 | 53 | 240.0 | 206.313514 | 216.0 | 144.0 | 26.433038 | 38168.0 | 698.705523 | 0 | 214 | ... | 24 | 56.137637 | 24.0 | 0.427521 | 0.999497 | -0.031726 | 0.031726 | 0.999497 | 6.941190 | 35.497670 |
53 | 54 | 248.0 | 224.251473 | 240.0 | 144.0 | 29.542436 | 114144.0 | 872.755534 | 221 | 215 | ... | 0 | 80.826651 | 0.0 | 0.000000 | 0.327038 | -0.945011 | 0.945011 | 0.327038 | 37.923490 | 43.556039 |
54 | 55 | 248.0 | 200.145985 | 208.0 | 128.0 | 27.374216 | 164520.0 | 749.347724 | 161 | 217 | ... | 0 | 108.157714 | 0.0 | 0.000000 | 0.119267 | -0.992862 | 0.992862 | 0.119267 | 41.764270 | 104.664511 |
55 | 56 | 232.0 | 195.920949 | 200.0 | 128.0 | 25.860310 | 49568.0 | 668.755631 | 131 | 224 | ... | 0 | 56.597713 | 0.0 | 0.000000 | 0.998990 | -0.044926 | 0.044926 | 0.998990 | 15.823917 | 25.661186 |
56 | 57 | 224.0 | 188.994924 | 192.0 | 136.0 | 24.472912 | 37232.0 | 598.923443 | 40 | 232 | ... | 0 | 50.123958 | 0.0 | 0.000000 | 0.989524 | 0.144369 | -0.144369 | 0.989524 | 11.684511 | 21.090000 |
57 | 58 | 216.0 | 184.761905 | 192.0 | 136.0 | 23.153557 | 15520.0 | 536.087206 | 118 | 249 | ... | 21 | 44.856208 | 21.0 | 0.468163 | 0.006588 | -0.999978 | 0.999978 | 0.006588 | 1.724907 | 28.033313 |
58 | 59 | 248.0 | 191.314286 | 184.0 | 144.0 | 30.233652 | 13392.0 | 914.073706 | 171 | 249 | ... | 17 | 37.271733 | 17.0 | 0.456110 | -0.007722 | -0.999970 | 0.999970 | -0.007722 | 1.757346 | 18.883470 |
59 | 60 | 248.0 | 204.600000 | 208.0 | 152.0 | 29.429446 | 8184.0 | 866.092308 | 228 | 250 | ... | 12 | 27.005740 | 12.0 | 0.444350 | -0.034966 | -0.999388 | 0.999388 | -0.034966 | 1.150116 | 9.234259 |
60 rows × 33 columns
These are all columns that are available:
print(statistics.keys())
Index(['label', 'maximum', 'mean', 'median', 'minimum', 'sigma', 'sum',
'variance', 'bbox_0', 'bbox_1', 'bbox_2', 'bbox_3', 'centroid_0',
'centroid_1', 'elongation', 'feret_diameter', 'flatness', 'roundness',
'equivalent_ellipsoid_diameter_0', 'equivalent_ellipsoid_diameter_1',
'equivalent_spherical_perimeter', 'equivalent_spherical_radius',
'number_of_pixels', 'number_of_pixels_on_border', 'perimeter',
'perimeter_on_border', 'perimeter_on_border_ratio', 'principal_axes0',
'principal_axes1', 'principal_axes2', 'principal_axes3',
'principal_moments0', 'principal_moments1'],
dtype='object')
df.describe()
label | maximum | mean | median | minimum | sigma | sum | variance | bbox_0 | bbox_1 | ... | number_of_pixels_on_border | perimeter | perimeter_on_border | perimeter_on_border_ratio | principal_axes0 | principal_axes1 | principal_axes2 | principal_axes3 | principal_moments0 | principal_moments1 | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
count | 60.000000 | 60.000000 | 60.000000 | 60.000000 | 60.000000 | 60.000000 | 60.000000 | 60.000000 | 60.000000 | 60.000000 | ... | 60.000000 | 60.000000 | 60.000000 | 60.000000 | 60.000000 | 60.000000 | 60.000000 | 60.000000 | 60.000000 | 60.000000 |
mean | 30.500000 | 236.000000 | 194.966598 | 200.800000 | 130.000000 | 26.069709 | 71696.933333 | 693.962389 | 123.516667 | 110.200000 | ... | 5.683333 | 107.224145 | 5.700000 | 0.089574 | 0.696028 | -0.138186 | 0.138186 | 0.696028 | 104.628848 | 134.931776 |
std | 17.464249 | 14.012101 | 16.394414 | 19.579304 | 7.456086 | 3.817798 | 41465.329504 | 183.657670 | 81.460709 | 76.672493 | ... | 10.255204 | 309.846236 | 10.310929 | 0.161164 | 0.476871 | 0.526743 | 0.526743 | 0.476871 | 651.200133 | 712.958883 |
min | 1.000000 | 176.000000 | 137.526132 | 136.000000 | 112.000000 | 13.360739 | 2576.000000 | 178.509343 | 0.000000 | 0.000000 | ... | 0.000000 | 14.843629 | 0.000000 | 0.000000 | -0.653319 | -0.999978 | -0.653870 | -0.653319 | 1.046852 | 1.755916 |
25% | 15.750000 | 232.000000 | 188.713774 | 192.000000 | 128.000000 | 24.472535 | 40604.000000 | 598.904954 | 52.500000 | 42.750000 | ... | 0.000000 | 53.221995 | 0.000000 | 0.000000 | 0.649215 | -0.663517 | -0.279282 | 0.649215 | 12.841678 | 22.351873 |
50% | 30.500000 | 240.000000 | 195.937410 | 200.000000 | 128.000000 | 26.409917 | 71016.000000 | 697.484271 | 127.000000 | 108.500000 | ... | 0.000000 | 68.482144 | 0.000000 | 0.000000 | 0.952820 | 0.026675 | -0.026675 | 0.952820 | 18.738377 | 35.597627 |
75% | 45.250000 | 248.000000 | 204.627083 | 210.000000 | 136.000000 | 28.649791 | 99386.000000 | 820.810634 | 201.500000 | 168.750000 | ... | 11.250000 | 83.278130 | 11.250000 | 0.142182 | 0.989550 | 0.279282 | 0.663517 | 0.989550 | 29.329379 | 56.857478 |
max | 60.000000 | 248.000000 | 229.430657 | 248.000000 | 152.000000 | 32.682072 | 172424.000000 | 1068.117840 | 251.000000 | 250.000000 | ... | 39.000000 | 2461.579651 | 40.000000 | 0.495128 | 0.999988 | 0.653870 | 0.999978 | 0.999988 | 5063.911496 | 5560.184284 |
8 rows × 33 columns
Specific measures#
SimpleITK offers some non-standard measurements which deserve additional documentation
perimeter_on_border_ratio
#
In this context, the SimpleITK documentation points to a publication which mentiones “‘SizeOnBorder’ is the number of pixels in the objects which are on the border of the image.” While the documentation is wage, the perimeter_on_border_ratio
may be related.
First, we check its range on the above example image.
perimeter_on_border_ratio = df['perimeter_on_border_ratio'].tolist()
np.min(perimeter_on_border_ratio), np.max(perimeter_on_border_ratio)
(0.0, 0.4951280471249033)
Next, we visualize the measurement in space.
perimeter_on_border_ratio_map = cle.replace_intensities(labels, [0] + perimeter_on_border_ratio)
perimeter_on_border_ratio_map
|
cle._ image
|
The visualization substantiates our presumption that the perimeter_on_border_ratio
indeed is related to the amount the object touches the image border.