Reading files with AICSImageIO#
The AICSImageIO library aims streamlining reading microscopy image data. There is also a napari plugin, but here we explain how to read it with the library.
To install the library, you need to call these commands.
mamba install aicsimageio bioformats_jar
In case some specific proprietary file formats fails to be read, additional software must be installed. Check the documentation for details.
For this notebook, we also need to install aicspylibczi to be able to read a .czi
file:
pip install aicspylibczi>=3.1.1 fsspec>=2022.8.0
from aicsimageio import AICSImage
from skimage.io import imshow
First, we create an AICSImage
object to see if it understands our file format. In the following we are using an image showing a Drosophila wing during pupal stage kindly provided by Romina Piscitello-Gómez (MPI CBG).
aics_image = AICSImage("../../data/PupalWing.czi")
aics_image
Attempted file (c:/Users/mazo260d/Documents/GitHub/PoL-BioImage-Analysis-TS-Early-Career-Track/data/PupalWing.czi) load with reader: aicsimageio.readers.czi_reader.CziReader failed with error: aicspylibczi is required for this reader. Install with `pip install 'aicspylibczi>=3.0.5' 'fsspec>=2022.7.1'`
<AICSImage [Reader: BioformatsReader, Image-is-in-Memory: False]>
This object can already give us basic information such as image size/shape, dimensions and dimension names and order.
aics_image.shape
(1, 1, 80, 520, 692)
aics_image.dims
<Dimensions [T: 1, C: 1, Z: 80, Y: 520, X: 692]>
aics_image.dims.order
'TCZYX'
From this object, we can also retrieve pixels as numpy arrays.
np_image = aics_image.get_image_data("ZYX", T=0)
np_image.shape
(80, 520, 692)
imshow(np_image[36])
c:\Users\mazo260d\mambaforge\envs\devbio-napari-env\lib\site-packages\skimage\io\_plugins\matplotlib_plugin.py:149: UserWarning: Low image data range; displaying image with stretched contrast.
lo, hi, cmap = _get_display_range(image)
<matplotlib.image.AxesImage at 0x12ddf24b670>
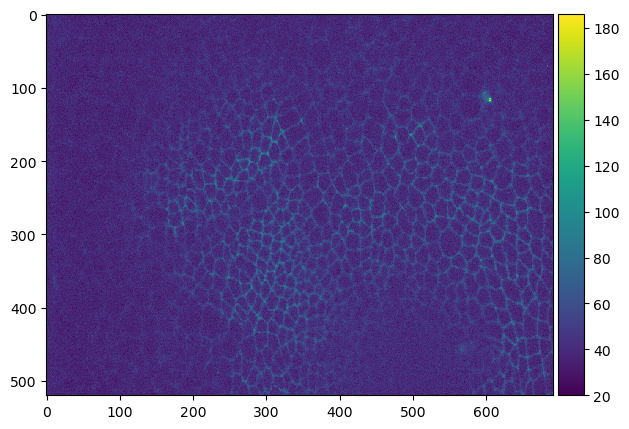
Reading meta data#
When working with microscopy image data, it is important to be aware of meta data, for example the voxel size. In order to do volume measurements in proper physical units, we need to know how large a voxel is in X, Y and Z.
aics_image.physical_pixel_sizes
PhysicalPixelSizes(Z=1.0, Y=0.20476190476190476, X=0.20476190476190476)
And one can define a helper function for reading the voxel size in Z/Y/X format.
def get_voxel_size_from_aics_image(aics_image):
return (aics_image.physical_pixel_sizes.Z,
aics_image.physical_pixel_sizes.Y,
aics_image.physical_pixel_sizes.X)
voxel_size = get_voxel_size_from_aics_image(aics_image)
voxel_size
(1.0, 0.20476190476190476, 0.20476190476190476)
Exercise#
Load this image to napari with the correct pixel sizes.
Hint: Set the scale
argument of the .add_image
method with the voxel size.